- Published on
Implementing Efficient Caching in JavaScript Using Map
- Authors
- Name
- Muyiwa Johnson
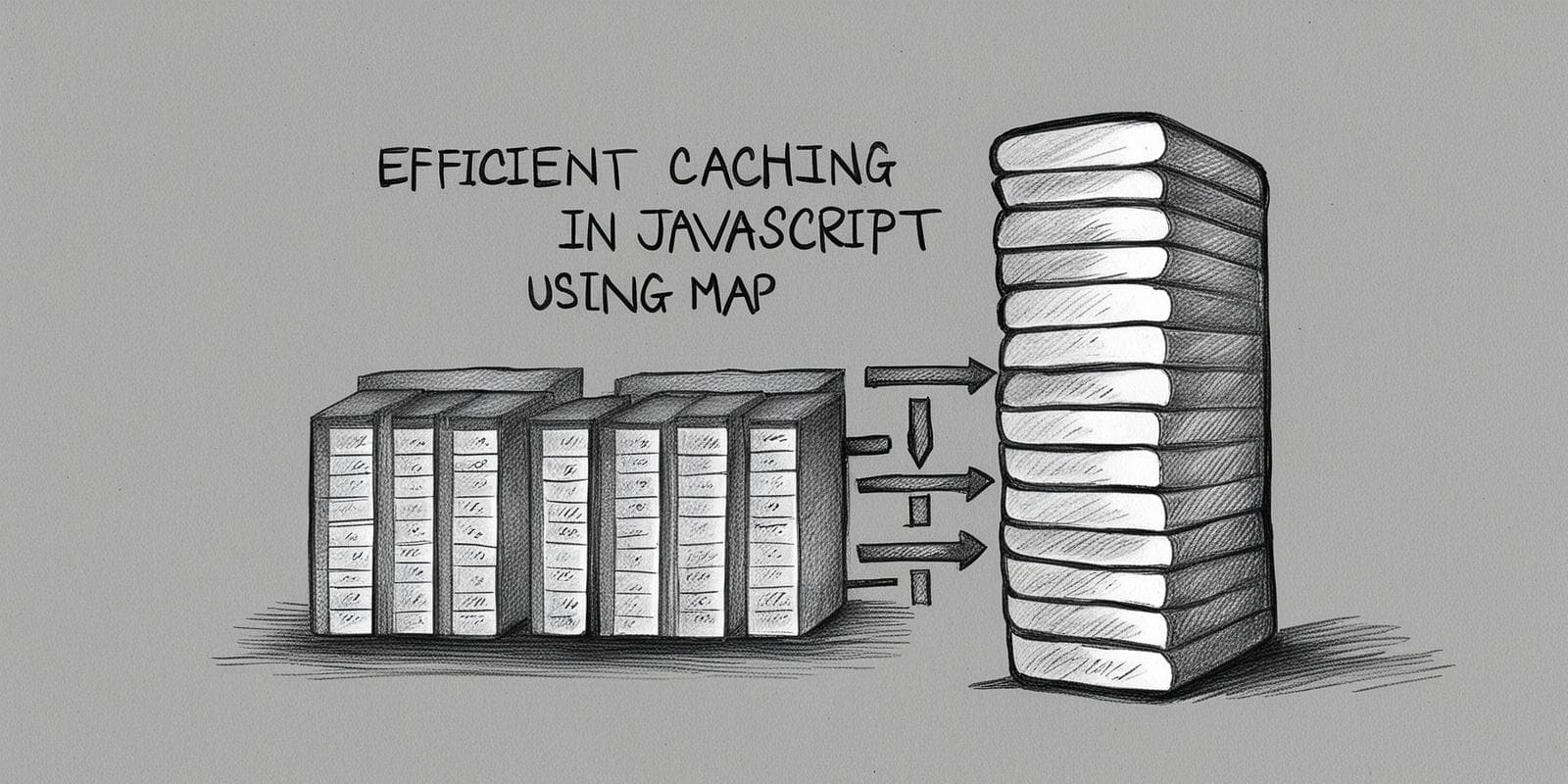
Introduction
Caching is a critical performance optimization technique that stores frequently accessed data in memory for faster retrieval. This guide demonstrates how to implement an efficient caching mechanism in JavaScript using the built-in Map
object.
Cache Implementation Fundamentals
Why Use Map for Caching?
The Map
object provides several advantages for caching:
- Key flexibility: Supports any value (objects or primitives) as keys
- Performance: O(1) time complexity for get/set operations
- Order preservation: Maintains insertion order (unlike plain objects)
- Built-in methods: Provides convenient cache management methods
Basic Cache Structure
// Initialize cache
const cache = new Map()
// Set cache entry
cache.set('user:42', { id: 42, name: 'Alice' })
// Get cache entry
const user = cache.get('user:42')
// Check existence
if (cache.has('user:42')) {
// Entry exists
}
// Delete entry
cache.delete('user:42')
// Clear entire cache
cache.clear()
Practical Implementation Patterns
1. Function Memoization
function memoize(fn) {
const cache = new Map()
return function (...args) {
const key = JSON.stringify(args)
if (cache.has(key)) {
return cache.get(key)
}
const result = fn(...args)
cache.set(key, result)
return result
}
}
// Usage
const factorial = memoize((n) => {
if (n <= 1) return 1
return n * factorial(n - 1)
})
2. API Response Caching
const apiCache = new Map()
const CACHE_TTL = 300000 // 5 minutes
async function getCachedData(url) {
const cached = apiCache.get(url)
if (cached && Date.now() - cached.timestamp < CACHE_TTL) {
return cached.data
}
const response = await fetch(url)
const data = await response.json()
apiCache.set(url, {
data,
timestamp: Date.now(),
})
return data
}
Cache Management Strategies
Expiration Policies
Policy | Implementation | Use Case |
---|---|---|
Time-based | Store timestamp with entries | API responses |
Size-based | Track cache size and prune | Memory-sensitive apps |
Manual | Explicit invalidation | Critical data |
Size-Limited Cache Implementation
class LRUCache {
constructor(maxSize = 100) {
this.cache = new Map()
this.maxSize = maxSize
}
get(key) {
if (!this.cache.has(key)) return undefined
const value = this.cache.get(key)
this.cache.delete(key)
this.cache.set(key, value)
return value
}
set(key, value) {
if (this.cache.size >= this.maxSize) {
const firstKey = this.cache.keys().next().value
this.cache.delete(firstKey)
}
this.cache.set(key, value)
}
}
Advanced Considerations
WeakMap Alternative
For memory-sensitive applications where cache entries should not prevent garbage collection:
const weakCache = new WeakMap()
const largeObject = {
/* ... */
}
weakCache.set(largeObject, computedValue)
// Entry automatically removed when largeObject is GC'd
Performance Characteristics
Operation | Time Complexity |
---|---|
set() | O(1) |
get() | O(1) |
has() | O(1) |
delete() | O(1) |
Best Practices
- Key Design: Create deterministic cache keys
- Expiration: Always implement cache expiration
- Monitoring: Track cache hit/miss ratios
- Size Limits: Prevent unbounded memory growth
- Invalidation: Clear stale data when source changes
Conclusion
The JavaScript Map
object provides an excellent foundation for building efficient caching solutions. By implementing proper cache management strategies and expiration policies, developers can significantly improve application performance while maintaining memory efficiency.
For production applications, consider these additional enhancements:
- Distributed cache support
- Persistent storage integration
- Advanced eviction policies
- Cache statistics collection